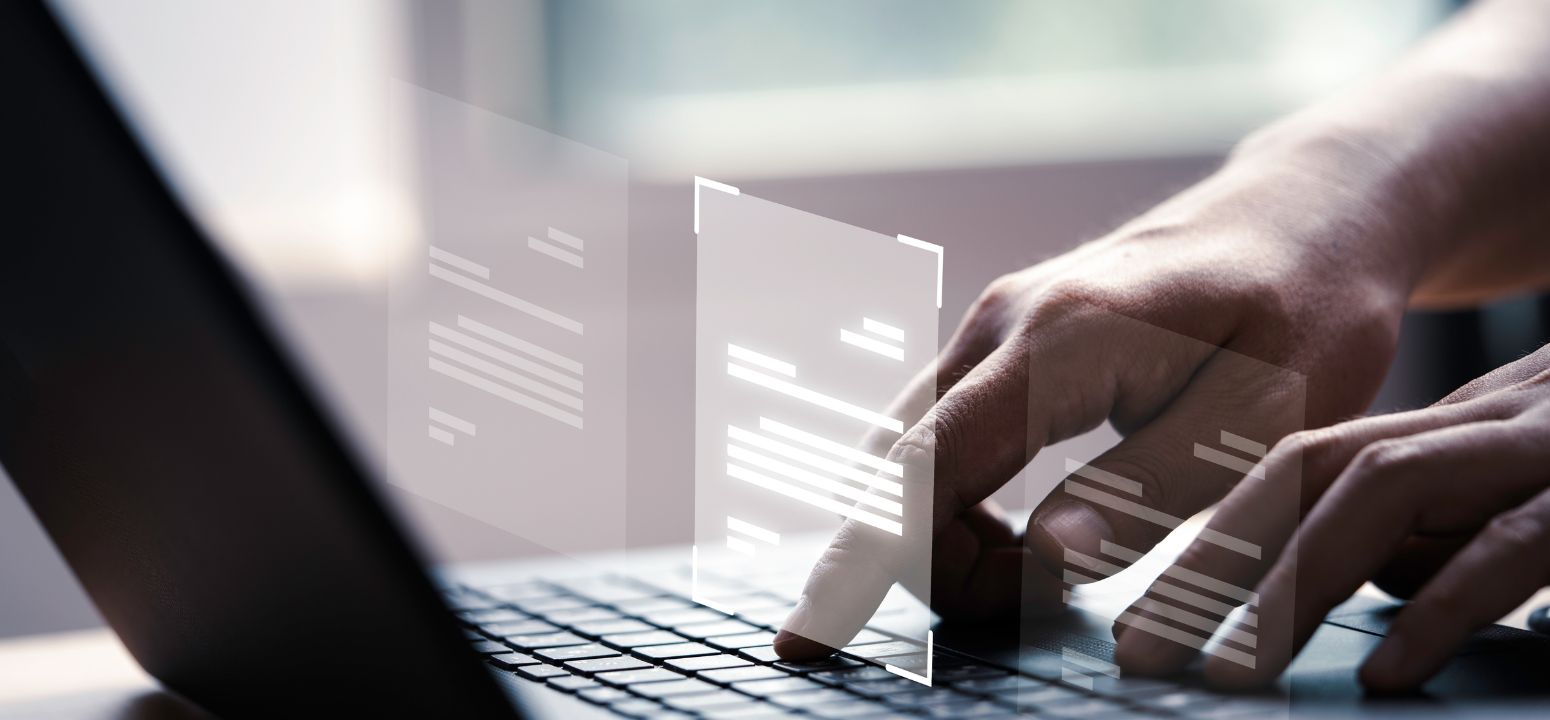
When developing UVM (Universal Verification Methodology) code, effective synchronization of simulation phases is critical. One of the most commonly used mechanisms for this synchronization is uvm_objection
, which ensures that a simulation does not exit a phase prematurely. However, improper or excessive use of objections can lead to inefficiencies, such as slower simulation times. This blog discusses practical ways to use objections effectively and explores alternative approaches for better efficiency and simplicity.
What is uvm_objection
?
UVM is built on processes that can be synchronized either automatically or manually across its phases. A common practice in UVM is to raise an objection at the beginning of a phase to prevent it from ending, and later drop the objection to allow the simulation to proceed. For instance, in the run phase, objections ensure that the simulation remains active until all objections have been dropped.
Here’s a simplified example:
systemverilogCopy code// Raising and dropping objections
initial begin
uvm_root.raise_objection(this);
#100; // Perform operations
uvm_root.drop_objection(this);
end
This mechanism is crucial when there are multiple components or sequences within the simulation, each with its unique tasks and timelines.
Challenges with Overusing Objections
In complex environments, multiple sequences and components might independently raise and drop objections. For instance, consider an environment with four sequences, each calling raise_objection
and drop_objection
. While functional, this approach introduces inefficiencies and can slow down the simulation.
Example of Inefficiency
systemverilogCopy codeforeach (env[i]) begin
env[i].raise_objection(this);
// Sequence operations
env[i].drop_objection(this);
end
As the simulation scales, excessive objections can create overhead, making the code harder to debug and maintain.
Efficient Alternatives to uvm_objection
1. Using uvm_barrier
for Synchronization
The uvm_barrier
class offers a lightweight alternative to objections. With only 240 lines of code, uvm_barrier
provides a mechanism to synchronize processes by counting down until all participants have completed their tasks.
Here’s an example of a custom barrier implementation:
systemverilogCopy codeclass my_barrier;
int count;
function new(int init_count);
count = init_count;
endfunction
task wait_barrier();
if (count > 0)
count--;
if (count == 0)
-> barrier_done; // Notify that the barrier is complete
endtask
endclass
This approach minimizes code and efficiently synchronizes processes, exiting as soon as the count reaches zero.
2. Named Synchronization Points
Another approach is to define synchronization points with descriptive names like start
, middle
, and end
. These points act as barriers within your simulation flow, ensuring clarity and structured synchronization.
systemverilogCopy codeclass sync_wrapper;
uvm_barrier barrier;
function new();
barrier = new(3); // Set the count for synchronization
endfunction
task synchronize(string phase);
if (phase == "start")
barrier.wait_barrier();
else if (phase == "middle")
barrier.wait_barrier();
else if (phase == "end")
barrier.wait_barrier();
endtask
endclass
This technique allows developers to clearly visualize and manage the synchronization of multiple phases, reducing clutter and improving maintainability.
Real-World Synchronization Example
Imagine a scenario where we have two SystemVerilog classes (class1
and class2
) and three Verilog modules (A
, B
, C
). Using named synchronization points (start
, middle
, end
), each object waits for the barrier to be passed before proceeding.
In each phase, the objects perform their operations, then drop the objection or decrement the barrier count, signaling readiness to move forward. Once all objects complete their phase, the simulation advances to the next phase.
Best Practices for Objection Usage
- Minimize the Use of
raise_objection
anddrop_objection
:
Limit their use to one or two critical points in the testbench. - Leverage Barriers for Lightweight Synchronization:
Useuvm_barrier
or custom implementations for simpler and faster synchronization. - Organize Code with Named Phases:
Descriptive names likestart
,middle
, andend
improve code readability and manageability. - Avoid Overlapping Objections:
Ensure that objections raised in one phase are properly dropped before raising new objections in subsequent phases.
Conclusion
uvm_objection
is a powerful tool for controlling simulation flow in UVM, but its excessive use can lead to inefficiencies. By incorporating lightweight alternatives such as uvm_barrier
or implementing custom synchronization techniques, you can simplify your code and improve simulation performance. Always strive for clean, efficient, and intuitive designs to make debugging and maintenance easier.
By following these practices, you can harness the full potential of UVM while keeping your simulations efficient and manageable.